Webprofilerbundleextra A Must Have Tool For Symfony2 Developers
Jul 4th, 2011When I started with Symfony2, I was confused by these kind of things I could read :
$em = $this->get('doctrine.orm.entity_manager');
$request = $this->get('request');
I could understand it when I was reading the code but I did not feel safe to reuse them some place else. I could not find any list of all the services I could call and I was not sure about what I would get by calling something. There is no reference or documentation for that.
WebProfilerBundleExtra is an enhancement of the debug toolbar of
Symfony2 that add some reference info by making list of stuff you can
use and debugging info. It is a bundle that you add to your project (and
load only in the dev
environment) that will add 4 tabs to the Web
Profiler displaying some precious info :
- Container : use this as a reference when developing, it lists all the parameters and service for the container that is used
- Routing : for routing info and debugging
- Twig : use this as a reference when developing, it lists all the extensions, tests filters and functions that you can use (loaded)
- Assetic : display assets collection
To install it, follow the instructions on the project home on github.
Container
If you are not familiar with containers in Symfony2, you should first read the Symfony2 book chapter Service Container (you can then go further with the Symfony2 Dependency Injection documentation). You will find in that tab two sections : Container Parameters and Container Services.
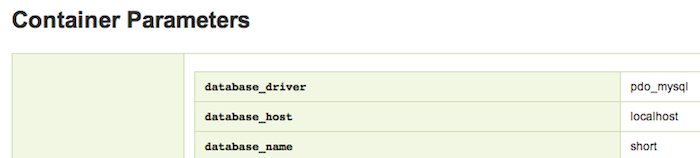
Container Parameters is the list of all the parameters that have
been defined in your applications. You can access parameters in your
controller by adding that it implements the interface
ContainerAwareInterface
:
use Symfony\Component\DependencyInjection\ContainerAwareInterface;
class PostController extends Controller implements ContainerAwareInterface {
public function indexAction() {
$container = $this->container;
$param = $container->getParameter('database_name'));
//...
}
}
Container Services are all the services you can access in you container.
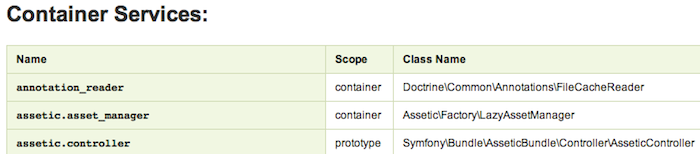
To access one service in a controller :
$em = $this->get('doctrine.orm.entity_manager');
Routing
You will find in that tab two sections : Sources and Routes. Sources is the list of all file that SF2 process to make the routing table. It’s a good start to debug if a route does not work: first check if the file in which you declare the route is processed by SF2.

Routes is the list of all the routes of your project. It gives you the same info than the command :
app/console router:debug
but add also the controller that goes which each route. It is a good way to have an overview of your routing for your app, as you define routes in a lot of different files.

Assetic
This tab display all the assets information of the assetic collections being used. Use that tabs when debugging problems with assets.
Twig
This tab is very useful when writing templates, use it as a reference. There is 4 sections : Twig Extensions, Twig Tests available, Twig Filters available and Twig Functions available. Twig Extensions is the list of all the Twig extensions loaded. Useful to debug when some filters or functions don’t work.
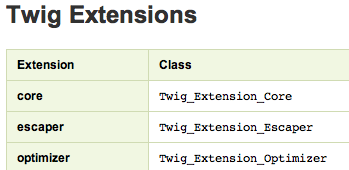
Twig Tests available is the list of the tests you can use in your Twig templates.
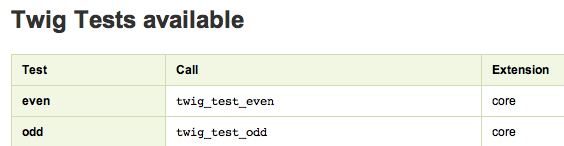
For example, to test if an number is divisible by 3 :
{% if loop.index is divisibleby(3) %}
Twig Filters available is the list of the filters you can use in your Twig templates.
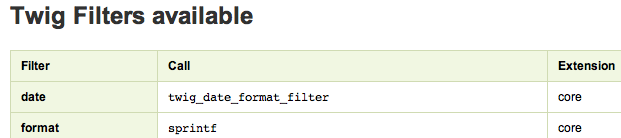
For example, to truncate a string :
{{ post.content | truncate(150) }}
Finally, Twig Functions available are the functions available.
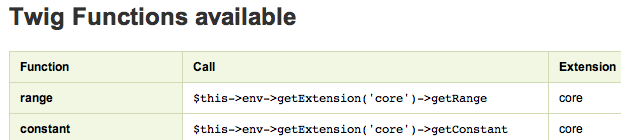
For example, the function range
to have a range :
{% for i in range(0, 3) %}